PINE LIBRARY
_matrix
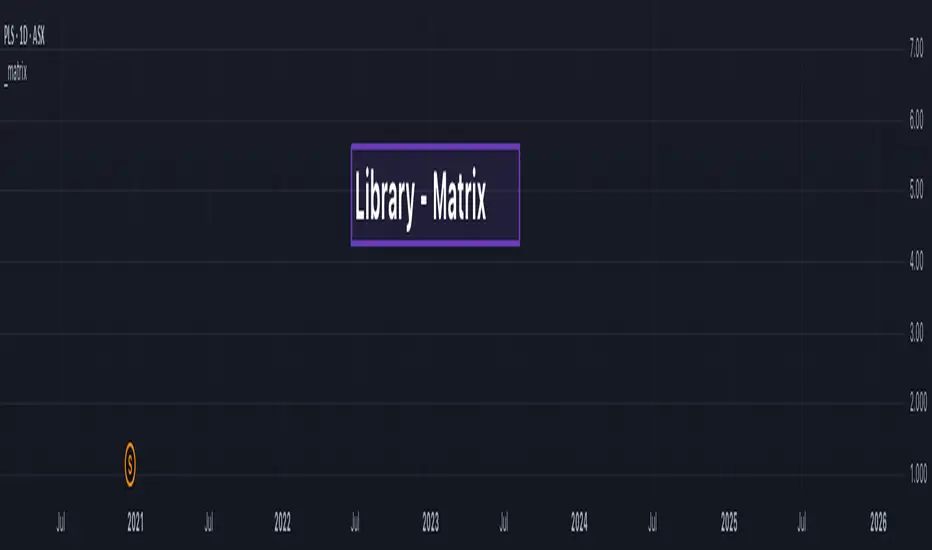
Library "_matrix"
Library helps visualize matrix as array of arrays and enables users to use array methods such as push, pop, shift, unshift etc along with cleanup activities on drawing objects wherever required
method delete(mtx, rowNumber)
deletes row from a matrix
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of objects
rowNumber (int): row index to be deleted
Returns: void
method delete(mtx, rowNumber)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
rowNumber (int)
method delete(mtx, rowNumber)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
rowNumber (int)
method delete(mtx, rowNumber)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
rowNumber (int)
method delete(mtx, rowNumber)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
rowNumber (int)
method delete(mtx, rowNumber)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
rowNumber (int)
method delete(mtx, rowNumber)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
rowNumber (int)
method delete(mtx, rowNumber)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
rowNumber (int)
method delete(mtx, rowNumber)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
rowNumber (int)
method delete(mtx, rowNumber)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
rowNumber (int)
method remove(mtx, rowNumber)
remove row from a matrix and returns them to caller
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of objects
rowNumber (int): row index to be deleted
Returns: type[]
method remove(mtx, rowNumber)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
rowNumber (int)
method remove(mtx, rowNumber)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
rowNumber (int)
method remove(mtx, rowNumber)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
rowNumber (int)
method remove(mtx, rowNumber)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
rowNumber (int)
method remove(mtx, rowNumber)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
rowNumber (int)
method remove(mtx, rowNumber)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
rowNumber (int)
method remove(mtx, rowNumber)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
rowNumber (int)
method remove(mtx, rowNumber)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
rowNumber (int)
method remove(mtx, rowNumber)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
rowNumber (int)
method unshift(mtx, row, maxItems)
unshift array of lines to first row of the matrix
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of lines
row (array<line>): array of lines to be inserted in row
maxItems (simple int)
Returns: resulting matrix of type
method unshift(mtx, row, maxItems)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
row (array<label>)
maxItems (simple int)
method unshift(mtx, row, maxItems)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
row (array<box>)
maxItems (simple int)
method unshift(mtx, row, maxItems)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
row (array<linefill>)
maxItems (simple int)
method unshift(mtx, row, maxItems)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
row (array<table>)
maxItems (simple int)
method unshift(mtx, row, maxItems)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
row (array<int>)
maxItems (simple int)
method unshift(mtx, row, maxItems)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
row (array<float>)
maxItems (simple int)
method unshift(mtx, row, maxItems)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
row (array<bool>)
maxItems (simple int)
method unshift(mtx, row, maxItems)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
row (array<string>)
maxItems (simple int)
method unshift(mtx, row, maxItems)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
row (array<color>)
maxItems (simple int)
method push(mtx, row, maxItems)
push array of lines to end of the matrix row
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of lines
row (array<line>): array of lines to be inserted in row
maxItems (simple int)
Returns: resulting matrix of lines
method push(mtx, row, maxItems)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
row (array<label>)
maxItems (simple int)
method push(mtx, row, maxItems)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
row (array<box>)
maxItems (simple int)
method push(mtx, row, maxItems)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
row (array<linefill>)
maxItems (simple int)
method push(mtx, row, maxItems)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
row (array<table>)
maxItems (simple int)
method push(mtx, row, maxItems)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
row (array<int>)
maxItems (simple int)
method push(mtx, row, maxItems)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
row (array<float>)
maxItems (simple int)
method push(mtx, row, maxItems)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
row (array<bool>)
maxItems (simple int)
method push(mtx, row, maxItems)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
row (array<string>)
maxItems (simple int)
method push(mtx, row, maxItems)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
row (array<color>)
maxItems (simple int)
method shift(mtx)
shift removes first row from matrix of lines
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of lines from which the shift operation need to be performed
Returns: void
method shift(mtx)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
method shift(mtx)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
method shift(mtx)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
method shift(mtx)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
method shift(mtx)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
method shift(mtx)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
method shift(mtx)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
method shift(mtx)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
method shift(mtx)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
method rshift(mtx)
rshift removes first row from matrix of lines and returns them as array
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of lines from which the rshift operation need to be performed
Returns: type[]
method rshift(mtx)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
method rshift(mtx)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
method rshift(mtx)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
method rshift(mtx)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
method rshift(mtx)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
method rshift(mtx)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
method rshift(mtx)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
method rshift(mtx)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
method rshift(mtx)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
method pop(mtx)
pop removes last row from matrix of lines
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of lines from which the pop operation need to be performed
Returns: void
method pop(mtx)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
method pop(mtx)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
method pop(mtx)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
method pop(mtx)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
method pop(mtx)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
method pop(mtx)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
method pop(mtx)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
method pop(mtx)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
method pop(mtx)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
method rpop(mtx)
rpop removes last row from matrix of lines and reutnrs the array to caller
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of lines from which the rpop operation need to be performed
Returns: void
method rpop(mtx)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
method rpop(mtx)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
method rpop(mtx)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
method rpop(mtx)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
method rpop(mtx)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
method rpop(mtx)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
method rpop(mtx)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
method rpop(mtx)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
method rpop(mtx)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
method clear(mtx)
clear clears the matrix
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of lines which needs to be cleared
Returns: void
method clear(mtx)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
method clear(mtx)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
method clear(mtx)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
method clear(mtx)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
method clear(mtx)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
method clear(mtx)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
method clear(mtx)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
method clear(mtx)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
method clear(mtx)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
method flush(mtx)
clear clears the matrix but retains the drawing objects
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of lines which needs to be cleared
Returns: void
method flush(mtx)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
method flush(mtx)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
method flush(mtx)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
method flush(mtx)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
method flush(mtx)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
method flush(mtx)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
method flush(mtx)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
method flush(mtx)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
method flush(mtx)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
Library helps visualize matrix as array of arrays and enables users to use array methods such as push, pop, shift, unshift etc along with cleanup activities on drawing objects wherever required
method delete(mtx, rowNumber)
deletes row from a matrix
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of objects
rowNumber (int): row index to be deleted
Returns: void
method delete(mtx, rowNumber)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
rowNumber (int)
method delete(mtx, rowNumber)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
rowNumber (int)
method delete(mtx, rowNumber)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
rowNumber (int)
method delete(mtx, rowNumber)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
rowNumber (int)
method delete(mtx, rowNumber)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
rowNumber (int)
method delete(mtx, rowNumber)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
rowNumber (int)
method delete(mtx, rowNumber)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
rowNumber (int)
method delete(mtx, rowNumber)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
rowNumber (int)
method delete(mtx, rowNumber)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
rowNumber (int)
method remove(mtx, rowNumber)
remove row from a matrix and returns them to caller
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of objects
rowNumber (int): row index to be deleted
Returns: type[]
method remove(mtx, rowNumber)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
rowNumber (int)
method remove(mtx, rowNumber)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
rowNumber (int)
method remove(mtx, rowNumber)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
rowNumber (int)
method remove(mtx, rowNumber)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
rowNumber (int)
method remove(mtx, rowNumber)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
rowNumber (int)
method remove(mtx, rowNumber)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
rowNumber (int)
method remove(mtx, rowNumber)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
rowNumber (int)
method remove(mtx, rowNumber)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
rowNumber (int)
method remove(mtx, rowNumber)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
rowNumber (int)
method unshift(mtx, row, maxItems)
unshift array of lines to first row of the matrix
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of lines
row (array<line>): array of lines to be inserted in row
maxItems (simple int)
Returns: resulting matrix of type
method unshift(mtx, row, maxItems)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
row (array<label>)
maxItems (simple int)
method unshift(mtx, row, maxItems)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
row (array<box>)
maxItems (simple int)
method unshift(mtx, row, maxItems)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
row (array<linefill>)
maxItems (simple int)
method unshift(mtx, row, maxItems)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
row (array<table>)
maxItems (simple int)
method unshift(mtx, row, maxItems)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
row (array<int>)
maxItems (simple int)
method unshift(mtx, row, maxItems)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
row (array<float>)
maxItems (simple int)
method unshift(mtx, row, maxItems)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
row (array<bool>)
maxItems (simple int)
method unshift(mtx, row, maxItems)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
row (array<string>)
maxItems (simple int)
method unshift(mtx, row, maxItems)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
row (array<color>)
maxItems (simple int)
method push(mtx, row, maxItems)
push array of lines to end of the matrix row
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of lines
row (array<line>): array of lines to be inserted in row
maxItems (simple int)
Returns: resulting matrix of lines
method push(mtx, row, maxItems)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
row (array<label>)
maxItems (simple int)
method push(mtx, row, maxItems)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
row (array<box>)
maxItems (simple int)
method push(mtx, row, maxItems)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
row (array<linefill>)
maxItems (simple int)
method push(mtx, row, maxItems)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
row (array<table>)
maxItems (simple int)
method push(mtx, row, maxItems)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
row (array<int>)
maxItems (simple int)
method push(mtx, row, maxItems)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
row (array<float>)
maxItems (simple int)
method push(mtx, row, maxItems)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
row (array<bool>)
maxItems (simple int)
method push(mtx, row, maxItems)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
row (array<string>)
maxItems (simple int)
method push(mtx, row, maxItems)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
row (array<color>)
maxItems (simple int)
method shift(mtx)
shift removes first row from matrix of lines
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of lines from which the shift operation need to be performed
Returns: void
method shift(mtx)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
method shift(mtx)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
method shift(mtx)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
method shift(mtx)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
method shift(mtx)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
method shift(mtx)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
method shift(mtx)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
method shift(mtx)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
method shift(mtx)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
method rshift(mtx)
rshift removes first row from matrix of lines and returns them as array
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of lines from which the rshift operation need to be performed
Returns: type[]
method rshift(mtx)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
method rshift(mtx)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
method rshift(mtx)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
method rshift(mtx)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
method rshift(mtx)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
method rshift(mtx)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
method rshift(mtx)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
method rshift(mtx)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
method rshift(mtx)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
method pop(mtx)
pop removes last row from matrix of lines
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of lines from which the pop operation need to be performed
Returns: void
method pop(mtx)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
method pop(mtx)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
method pop(mtx)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
method pop(mtx)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
method pop(mtx)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
method pop(mtx)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
method pop(mtx)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
method pop(mtx)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
method pop(mtx)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
method rpop(mtx)
rpop removes last row from matrix of lines and reutnrs the array to caller
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of lines from which the rpop operation need to be performed
Returns: void
method rpop(mtx)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
method rpop(mtx)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
method rpop(mtx)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
method rpop(mtx)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
method rpop(mtx)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
method rpop(mtx)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
method rpop(mtx)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
method rpop(mtx)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
method rpop(mtx)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
method clear(mtx)
clear clears the matrix
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of lines which needs to be cleared
Returns: void
method clear(mtx)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
method clear(mtx)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
method clear(mtx)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
method clear(mtx)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
method clear(mtx)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
method clear(mtx)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
method clear(mtx)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
method clear(mtx)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
method clear(mtx)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
method flush(mtx)
clear clears the matrix but retains the drawing objects
Namespace types: matrix<line>
Parameters:
mtx (matrix<line>): matrix of lines which needs to be cleared
Returns: void
method flush(mtx)
Namespace types: matrix<label>
Parameters:
mtx (matrix<label>)
method flush(mtx)
Namespace types: matrix<box>
Parameters:
mtx (matrix<box>)
method flush(mtx)
Namespace types: matrix<linefill>
Parameters:
mtx (matrix<linefill>)
method flush(mtx)
Namespace types: matrix<table>
Parameters:
mtx (matrix<table>)
method flush(mtx)
Namespace types: matrix<int>
Parameters:
mtx (matrix<int>)
method flush(mtx)
Namespace types: matrix<float>
Parameters:
mtx (matrix<float>)
method flush(mtx)
Namespace types: matrix<bool>
Parameters:
mtx (matrix<bool>)
method flush(mtx)
Namespace types: matrix<string>
Parameters:
mtx (matrix<string>)
method flush(mtx)
Namespace types: matrix<color>
Parameters:
mtx (matrix<color>)
مكتبة باين
كمثال للقيم التي تتبناها TradingView، نشر المؤلف شيفرة باين كمكتبة مفتوحة المصدر بحيث يمكن لمبرمجي باين الآخرين من مجتمعنا استخدامه بحرية. تحياتنا للمؤلف! يمكنك استخدام هذه المكتبة بشكل خاص أو في منشورات أخرى مفتوحة المصدر، ولكن إعادة استخدام هذا الرمز في المنشورات تخضع لقواعد الموقع.
Trial - trendoscope.io/trial
Subscribe - trendoscope.io/pricing
Blog - docs.trendoscope.io
Subscribe - trendoscope.io/pricing
Blog - docs.trendoscope.io
إخلاء المسؤولية
لا يُقصد بالمعلومات والمنشورات أن تكون، أو تشكل، أي نصيحة مالية أو استثمارية أو تجارية أو أنواع أخرى من النصائح أو التوصيات المقدمة أو المعتمدة من TradingView. اقرأ المزيد في شروط الاستخدام.
مكتبة باين
كمثال للقيم التي تتبناها TradingView، نشر المؤلف شيفرة باين كمكتبة مفتوحة المصدر بحيث يمكن لمبرمجي باين الآخرين من مجتمعنا استخدامه بحرية. تحياتنا للمؤلف! يمكنك استخدام هذه المكتبة بشكل خاص أو في منشورات أخرى مفتوحة المصدر، ولكن إعادة استخدام هذا الرمز في المنشورات تخضع لقواعد الموقع.
Trial - trendoscope.io/trial
Subscribe - trendoscope.io/pricing
Blog - docs.trendoscope.io
Subscribe - trendoscope.io/pricing
Blog - docs.trendoscope.io
إخلاء المسؤولية
لا يُقصد بالمعلومات والمنشورات أن تكون، أو تشكل، أي نصيحة مالية أو استثمارية أو تجارية أو أنواع أخرى من النصائح أو التوصيات المقدمة أو المعتمدة من TradingView. اقرأ المزيد في شروط الاستخدام.